Learning C# by Programming Games
Learning C# by Programming Games(2019)
摘要
class GameState From that point on, it will be forbidden to create a new instance of the GameState class. Try to write new GameState() somewhere (e.g., in the LoadContent method of PenguinPairs) and you’ll see that the compiler doesn’t allow it anymore. At the same time, you can still create instances of all subclasses of GameState, because those subclasses are not abstract. When you see this for the first time, you may wonder why abstract classes are useful. Why can’t programmers just pinky-promise that they won’t create any GameStateinstances? Well, yes, that’s also possible.1 Abstract classes are not required in a program. However, you can use abstract classes to make a program much clearer for other programmers. If a programmer sees the keyword abstract, they’ll immediately know what they’re dealing with: a class of which they cannot make new instances. Also, instead of having to check yourself that all programmers keep their promises, it’s safer and easier to let the compiler take care of that. By the way, abstract classes are not exclusive to C#: they’re also supported by many other objectoriented languages, such as Java and C++. 1Although we’re not sure if a pinky-promise has any legal value. 17.3 Abstract Classes 339 17.3.2 Abstract Classes in the Game Engine Next to the GameState class, your game engine contains a few other classes that you can mark as abstract. These are the classes of which you’ll never create any instances, but that you’ll definitely need subclasses of. Can you guess which ones they are? There are two of these classes in the Engine folder: ExtendedGame and GameObject. Every game will have a main class that inherits from ExtendedGame, but the main class will never be ExtendedGame itself. Also, each game object will either be a SpriteGameObject, a TextGameObject, or a GameObjectList. You will never create an instance of GameObject itself, simply because it doesn’t provide enough functionality on its own. In the PenguinPairs1b example project (and all project from this point on), we’ve made these classes abstract. Way back in Painter game, the ThreeColorGameObject class was also a good candidate for becoming abstract. After all, we never created any instances of that class itself, but only of its subclasses (such as Ball and PaintCan). 17.3.3 Abstract Methods You can go even further with abstract classes, though. Within an abstract class, you can also add abstract methods. Unlike the methods you’ve seen so far, an abstract method is a method without a body. By adding such a method to your class, you will promise that the method exists (and with which parameters), but you don’t yet have to specify what it actually does. To live up to your promise, all subclasses of your abstract class must then implement this method. This is useful if you know that multiple subclasses need to do the same type of task but that the details of this task will be complete different for each class. For example, imagine a game with the following abstract class: abstract class Animal { public void Speak(); } This says that all animals in the game should be able to speak, without giving any further details. Any class that inherits from Animal must then implement the Speak method: class Dog : Animal { public override void Speak() { Console.WriteLine(”Woof!”); } } Just like with normal inheritance, you use the keyword override to tell the compiler that this Speak method is indeed a more specific version of Animal’s Speak method. A (non-abstract) class is only valid if it implements all abstract methods of its parent class. Of course, a child class of an abstract class can itself be abstract again. In that case, it doesn’t yet have to implement all abstract methods. Note: Abstract methods are only allowed inside abstract classes. In other words, a class with at least one abstract method must be an abstract class. However, an abstract class is also allowed to have zero abstract methods. The GameState class is currently an example of this. 340 17 Better Game State Management In our game engine, we don’t have to add any abstract methods yet. However, you now at least know about them, and we expect that you’ll want to use them soon enough in games of your own. 17.3.4 Abstract Properties Next to abstract methods, an abstract class can also have abstract properties. In that case, you only specify whether the property has get and set components, but you don’t yet specify what those components do. Here are two examples: protected abstract bool MyReadOnlyProperty { get; } protected abstract int MyOtherProperty { get; set; } And again, subclasses can fill in these abstract properties using the keyword override: protected override bool MyReadOnlyProperty { get { ... } } protected override int MyOtherProperty { get { ... } set { ... } } You won’t need abstract properties anytime soon in this book, but it’s good to know that the possibility exists. Quick Reference: Abstract Classes If you add the keyword abstract to the header of a class MyClass: abstract class MyClass { ... } then that class will be abstract. This means that it’s forbidden to create an instance of MyClass anywhere in your code (by writing new MyClass(...)). However, you’re still allowed to create instances of subclasses of MyClass. This is useful if MyClass describes code shared by other classes, but doesn’t describe a concrete object yet. An abstract class can also have abstract methods (and properties). Such a method only has a header and no body: public abstract void MyAbstractMethod(); Subclasses of MyClass can then implement this method, using the keyword override as usual: public override void DoSomething() { ... } If a non-abstract class A inherits from an abstract class B, then A is only valid if it implements all abstract methods and properties of B.
更多查看译文
AI 理解论文
溯源树
样例
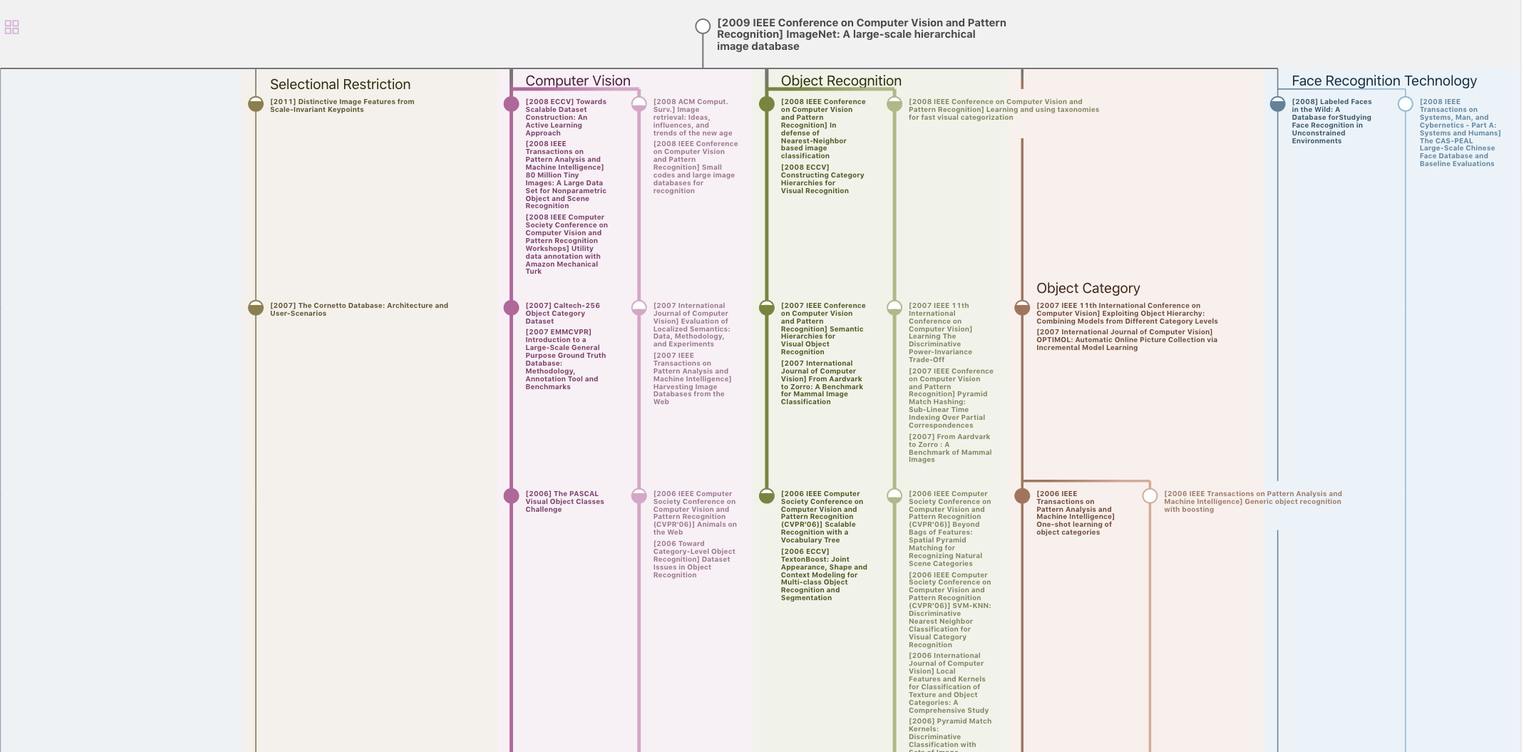
生成溯源树,研究论文发展脉络
Chat Paper
正在生成论文摘要